bsl.StreamReceiver¶
- class bsl.StreamReceiver(bufsize=1, winsize=1, stream_name=None)[source]¶
Class for data acquisition from LSL streams.
- It supports the streams of:
EEG
Markers
- Parameters:
- bufsize
int
|float
Buffer’s size
[secs]
.MAX_BUF_SIZE
(def: 1-day) is the maximum size. Large buffer may lead to a delay if not pulled frequently.- winsize
int
|float
Window’s size
[secs]
. Must be smaller than the buffer’s size.- stream_name
list
|str
|None
Servers’ name or list of servers’ name to connect to. If
None
, connects to all the available streams.
- bufsize
Attributes
Buffer's size
[sec]
.Connected status.
Dictionary containing the Info for the compatible streams.
Connected stream's name.
Connected streams dictionary
{stream_name: _Stream}
.Window's size
[sec]
.Methods
acquire
()Read data from the streams and fill their buffer using threading.
connect
([stream_name, timeout, force])Connect to the LSL streams.
disconnect
([stream_name])Disconnect the stream
stream_name
from the StreamReceiver.get_buffer
([stream_name, return_raw])Get the entire buffer of a stream.
get_window
([stream_name, return_raw])Get the latest window from a stream's buffer.
reset_buffer
([stream_name])Clear the stream's buffer.
Display the information about the connected streams.
- connect(stream_name=None, timeout=5, force=False)[source]¶
Connect to the LSL streams.
Search for the available streams on the LSL network and connect to the appropriate ones. If a LSL stream fulfills the requirements (name…), a connection is established.
This function is called while instantiated a StreamReceiver and can be recall to reconnect to the LSL streams.
- Parameters:
- Returns:
- success
bool
True if the connection was successful.
- success
- disconnect(stream_name=None)[source]¶
Disconnect the stream
stream_name
from the StreamReceiver.If
stream_name
is alist
, disconnects all streams in the list. Ifstream_name
isNone
, disconnects all streams.
- get_buffer(stream_name=None, return_raw=False)[source]¶
Get the entire buffer of a stream.
If several streams are connected, specify the name.
- Parameters:
- stream_name
str
|None
Name of the stream from which data is retrieved. Can be set to
None
(default) if the StreamReceiver is connected to a single stream.- return_raw
bool
By default (
False
), data is returned as aarray
of shape(samples, channels)
. If set toTrue
, the StreamReceiver will attempt to return data as a MNE Raw instances.
- stream_name
- Returns:
Notes
Returns a raw data array in the unit streamed by the LSL outlet. For conversion the corresponding scaling factor must be set for each stream, with e.g. for a stream in uV to convert to V:
sr.streams['stream_to_convert'].scaling_factor = 1e-6
- get_window(stream_name=None, return_raw=False)[source]¶
Get the latest window from a stream’s buffer.
If several streams are connected, specify the name.
- Parameters:
- stream_name
str
|None
Name of the stream from which data is retrieved. Can be set to
None
(default) if the StreamReceiver is connected to a single stream.- return_raw
bool
By default (
False
), data is returned as aarray
of shape(samples, channels)
. If set toTrue
, the StreamReceiver will attempt to return data as a MNE Raw instances.
- stream_name
- Returns:
Notes
Returns a raw data array in the unit streamed by the LSL outlet. For conversion the corresponding scaling factor must be set for each stream, with e.g. for a stream in uV to convert to V:
sr.streams['stream_to_convert'].scaling_factor = 1e-6
Examples using bsl.StreamReceiver
¶
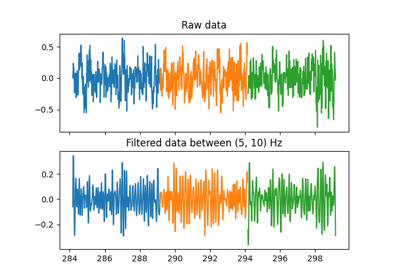
StreamReceiver: real-time buffer filtered with a causal filter